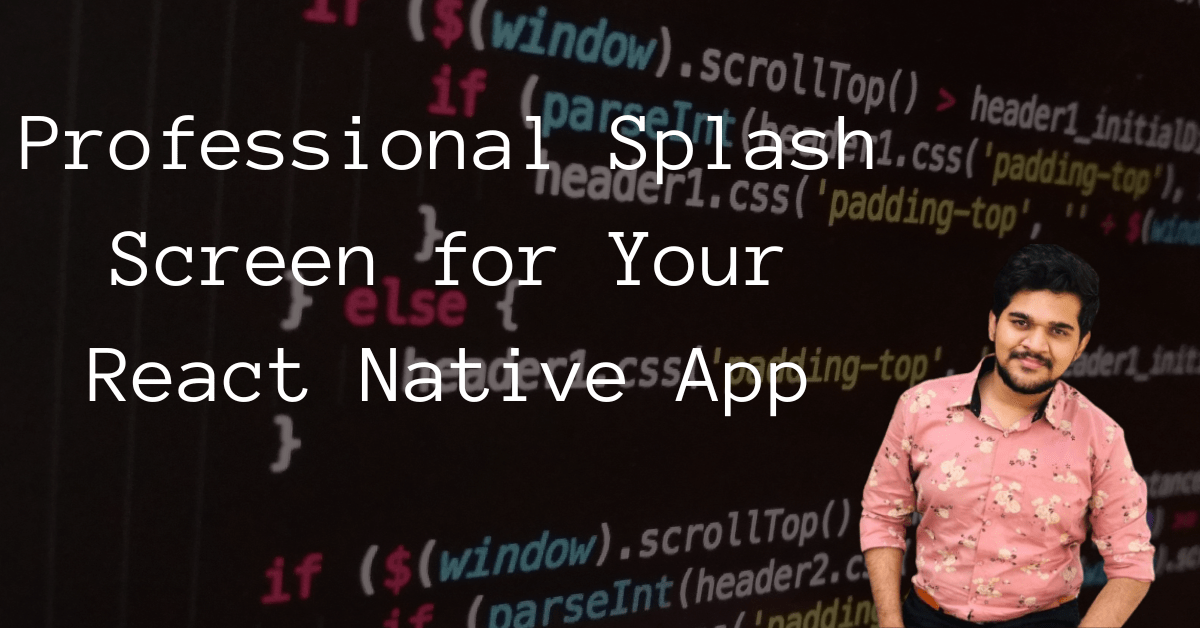
Professional Splash Screen for Your React Native App
There are 2 methods we can create splash screen
- React native splash screen through component
- React native splash screen through native code
React native splash screen through component
Creating a splash screen for a React Native application is a straightforward process. Here are the basic steps:
- Create a new image asset that will be used as your splash screen. The image should be in PNG or JPEG format and have a size that is appropriate for the device resolutions that your app supports.
- Add the image to your React Native project by placing it in the project’s ‘src/assets’ directory.
- Create a new component in your project’s ‘src’ directory, for example, ‘SplashScreen.js’.
- In the new component, import the image asset using the following code:
import React from 'react';
import { Image, StyleSheet, View } from 'react-native';
import splashImage from '../assets/splash.png';
- Use the imported image in your component’s render method:
export default function SplashScreen() {
return (
<View style={styles.container}>
<Image source={splashImage} style={styles.image} />
</View>
);
}
- Finally, add the SplashScreen component to your application’s root component, for example, App.js, by rendering it before the rest of the application’s content:
import React from 'react';
import { View, Text } from 'react-native';
import SplashScreen from './src/SplashScreen';
export default function App() {
return (
<View>
<SplashScreen />
<Text>Welcome to my app!</Text>
{/* Add more content here */}
</View>
);
}
By following these steps, you can create a simple splash screen for your React Native application. You can customize the splash screen by changing the image asset and the styles applied to it.
React native splash screen through Native code
Yes, you can also add a splash screen through native code in React Native. Here are the basic steps:
- In the project’s native code, create a new activity that will display the splash screen. For example, in Android, you can create a new activity class called SplashScreenActivity.
- In the new activity’s onCreate method, set the content view to your splash screen layout. The layout can be a simple image or a more complex layout that includes animations or other elements.
- Set the theme for your application to your splash screen theme in the AndroidManifest.xml file:
<application
android:name=".MainApplication"
android:label="@string/app_name"
android:theme="@style/SplashScreenTheme">
- In iOS, create a new LaunchScreen.storyboard file in Xcode and design the splash screen using Interface Builder.
- In the LaunchScreen.storyboard file, set the initial view controller to your splash screen view controller.
- Build and run your application. The splash screen will now be displayed while the app is loading.
By following these steps, you can add a splash screen through native code in both Android and iOS for your React Native application. Keep in mind that this method may require more setup and configuration than creating a splash screen using React Native components, but it can give you more flexibility in designing your splash screen.