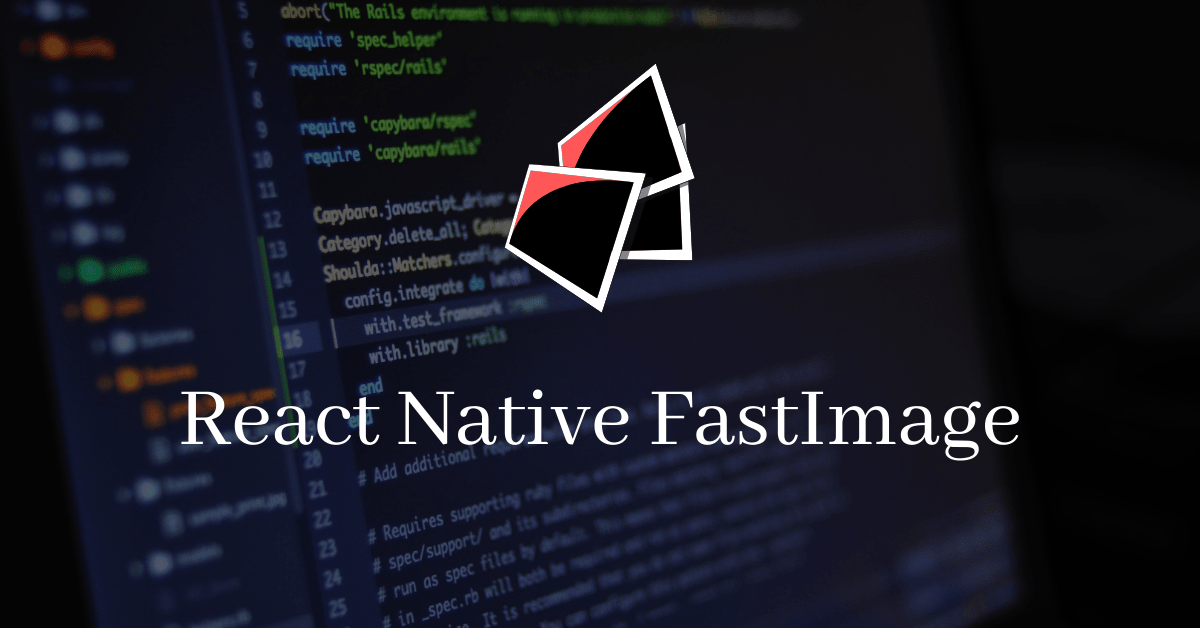
React Native FastImage
React Native Image component has some issues. That lack some performance. Caching is not working fine and many more. That’s why we need to use react native fastimage.
Installation
using npm
npm i react-native-fast-image --save
using yarn
yarn add react-native-fast-image
Usage of React Native FastImage
Firstly import the library at the top
import FastImage from 'react-native-fast-image'
Now comes the fastimage component
const YourImage = () => (
<FastImage
style={{ width: 200, height: 200 }}
source={{
uri: 'https://unsplash.it/400/400?image=1',
headers: { Authorization: 'someAuthToken' },
priority: FastImage.priority.normal,
}}
resizeMode={FastImage.resizeMode.contain}
/>
)
Important useful Properties (props)
Now comes the most important part. For implementing this we need to use all important props according to our need. So some important once are here.
1. Source –
It is a object which takes url, header, cache and all important values. Everything we need is under this source object.
uri | The url of the image which we want to show. |
headers | Headers to load the image. |
priority | Here we give the priority to low, normal or high. Default value is normal (FastImage.priority.normal). |
cache | FastImage.cacheControl.immutable – (Default) – Only updates if url changes.FastImage.cacheControl.web – Use headers and follow normal caching procedures.FastImage.cacheControl.cacheOnly – Only show images from cache, do not make any network requests. |
resizeMode
We all use different type of image in our app. All are from different dimensions then we can resize that according to our usage. These are the possible options.
FastImage.resizeMode.contain
– Scale the image uniformly (maintain the image’s aspect ratio) so that both dimensions (width and height) of the image will be equal to or less than the corresponding dimension of the view (minus padding).FastImage.resizeMode.cover
(Default) – Scale the image uniformly (maintain the image’s aspect ratio) so that both dimensions (width and height) of the image will be equal to or larger than the corresponding dimension of the view (minus padding).FastImage.resizeMode.stretch
– Scale width and height independently, This may change the aspect ratio of the src.FastImage.resizeMode.center
– Do not scale the image, keep centered.
style
The react native style property
If you have any other question or problem comment down below. I am happy to answer you.